Converting my favourite PHP functions to JS Part 2 - array_chunk
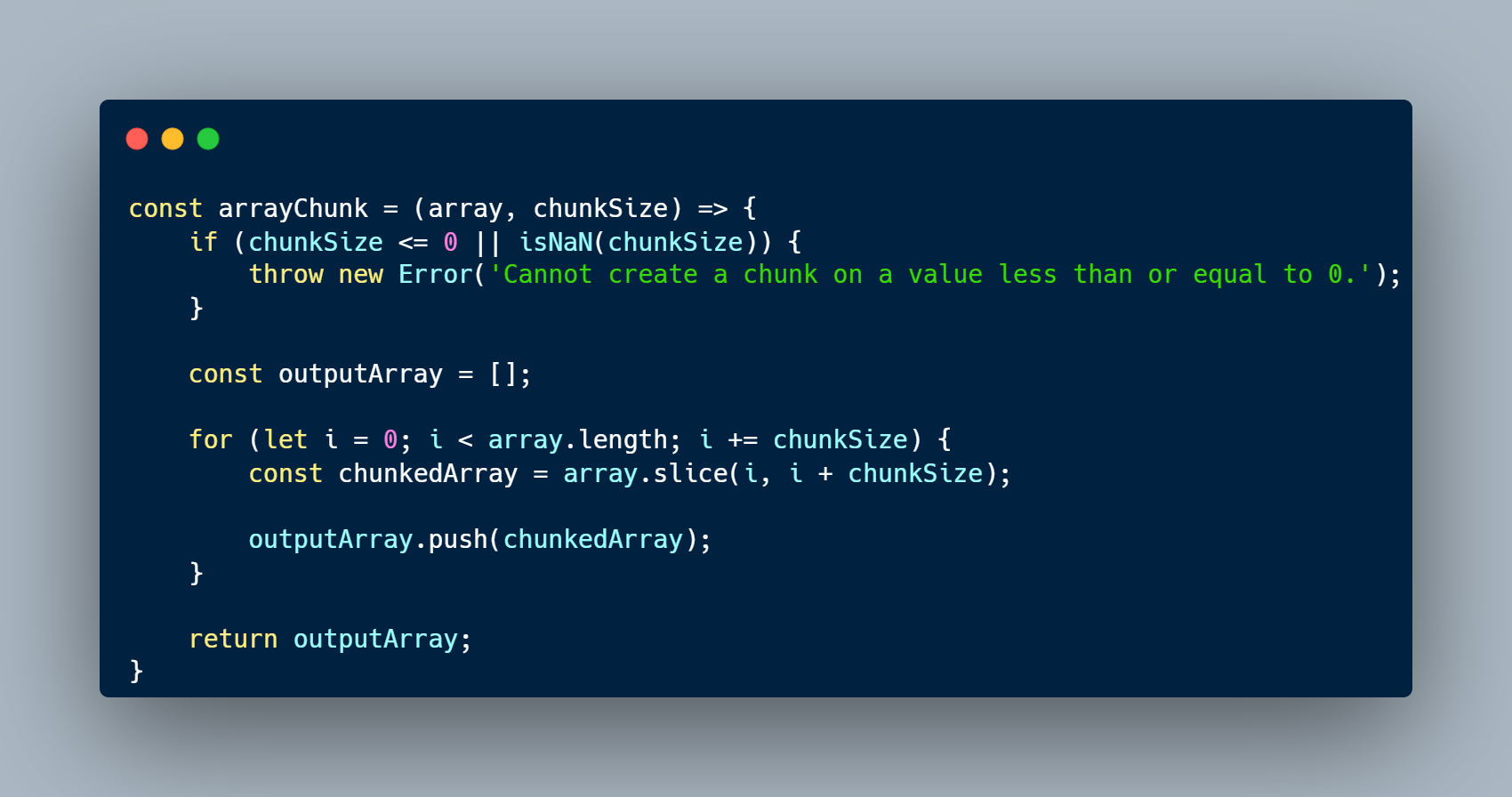
In this part, we're going to create the array_chunk method.
Simply put - array_chunk() will take in an array, and a chunk size. It will then return an array of arrays, where each array has been split down to the size you set in the chunk size. Make sense?
For example:
arrayChunk([1,2,3,4,5,6,7,8,9,10], 2);
Should give the following return value:
[ [ 1, 2 ], [ 3, 4 ], [ 5, 6 ], [ 7, 8 ], [ 9, 10 ] ]
This is a fairly simple function to write - I'll post the final copy here and go over it afterwards.
Firstly - we want to ensure that the user has input a number that's greater than 0. If they haven't, we'll throw a new error.
The main logic is in our for loop. We want to iterate our original array, and on each iteration we want to increment i by the chunkSize parameter passed into the method.
We create a chunk out of the array by simply slicing the original array, this returns to us a new array from 2 indexes. For example, the first iteration would be array.slice(0, 2), then array.slice(2, 4) etc. The slice method always goes up to, but not including, the index you pass as the second parameter.
When we've sliced the array, we simply push it into an outputArray variable, which will be our return value.
Use Case
My main use case for this function in PHP was in situations where I wanted to do mass updates or inserts to the database, but only certain amounts at a time.